Memory management is often a complex issue in a system written in a language without garbage collection. The situation is mostly simple in PBRT since most dynamic memory allocation is done as the scene description file is parsed, and most of this memory remains in use until rendering is finished. Nevertheless, there are a few issues related to memory management most of them performance related that warrant classes and utility routines to address them.
Main memory (RAM) is the primary memory, which a CPU can access directly. Therefore, any instruction in execution and data that is used by instruction must be in one of the direct storage access devices (RAM).
Memories are made up of registers. Each register in the memory is one storage location. The storage location is also called a memory location. Memory locations are identified using Address. The total number of bits a memory can store is its capacity.
- Memory management is concerned with managing primary memory.
- Memory consists of an array of bytes or words each with its own address.
- The instructions are fetched from the memory by the CPU based on the value program counter. These instructions may cause additional loading from and storing to specific memory addresses.
- The memory unit sees only a stream of memory addresses. It does not know how they are generated.
- The program must be brought into memory and placed within a process for it to be run.
- Input queue: a collection of processes on the disk that are waiting to be brought into memory for execution.
- User programs go through several steps before being run.
Functions of memory management:
- Keeping track of the status of each memory location.
- Determining the allocation policy – Memory allocation technique & Deallocation technique.
Address Binding:
- Programs are stored on the secondary storage disks as binary executable files.
- When the programs are to be executed they are brought into the main memory and placed within a process.
- The collection of processes on the disk waiting to enter the main memory forms the input queue.
- One of the processes which are to be executed is fetched from the queue and placed in the main memory.
- During the execution, it fetches instructions and data from the main memory. After the process terminates it returns back the memory space.
- During execution, the process will go through different steps and in each step, the address is represented in different ways.
- In the source program, the address is symbolic.
- The compiler converts the symbolic address to a re-locatable address.
- The loader will convert this re-locatable address to an absolute address.
Binding of instructions and data can be done at any step along the way:
- Compile time: If we know whether the process resides in memory then absolute code can be generated. If the static address changes then it is necessary to re-compile the code from the beginning.
- Load time: If the compiler doesn’t know whether the process resides in memory then it generates the re-locatable code. In this, the binding is delayed until the load time.
- Execution time: If the process is moved during its execution from one memory segment to another then the binding is delayed until run time. Special hardware is used for this. The most general-purpose operating system uses this method.
Logical and Physical address map:
- The address generated by the CPU is called a logical address or virtual address.
- The address seen by the memory unit i.e., the one loaded into the memory register is called the physical address.
- Compile time and load time address binding methods generate some logical and physical addresses. The execution time address binding generates the different logical and physical addresses.
- The set of logical address spaces generated by the programs is the logical address space.
- The set of the physical address corresponding to these logical addresses is the physical address space.
- The mapping of the virtual addresses to physical addresses during run time is done by the hardware device called a memory management unit (MMU).
- The base register is also called the re-location register. The value of the relocation register is added to every address generated by the user
- The process at the time it is sent to memory.
- The user program never sees the real physical address.
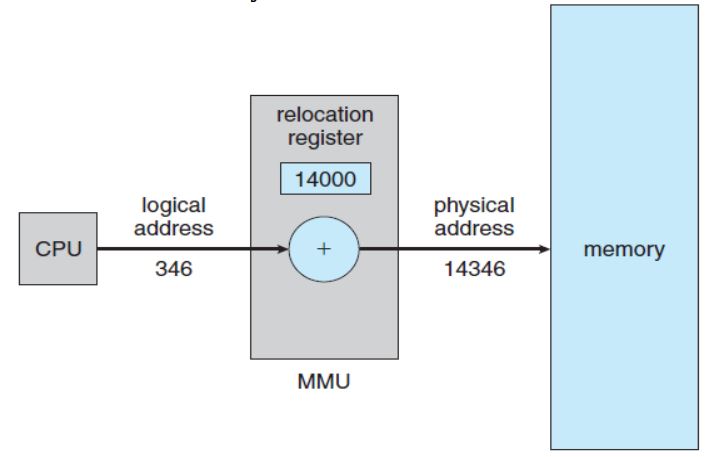
Dynamic Loading:
- For a process to be executed it should be loaded into the physical memory. The size of the process is limited to the size of the physical memory.
- Dynamic loading is used to obtain better memory utilization.
- In dynamic loading, the routine or procedure will not be loaded until it is called.
- Whenever a routine is called, the calling routine first checks whether the called routine is already loaded or not. If it is not loaded it cause the loader to load the desired program into the memory and updates the program’s address table to indicate the change and control is passed to the newly called routine.
Advantage:
- Gives better memory utilization.
- An unused routine is never loaded.
- Do not need special operating system support.
- This method is useful when large amounts of codes are needed to handle frequently occurring cases.
Swapping:
Swapping is a technique of temporarily removing inactive programs from the memory of the system. A process can be swapped temporarily out of the memory to a backing store and then brought back into the memory for continuing the execution. This process is called swapping.
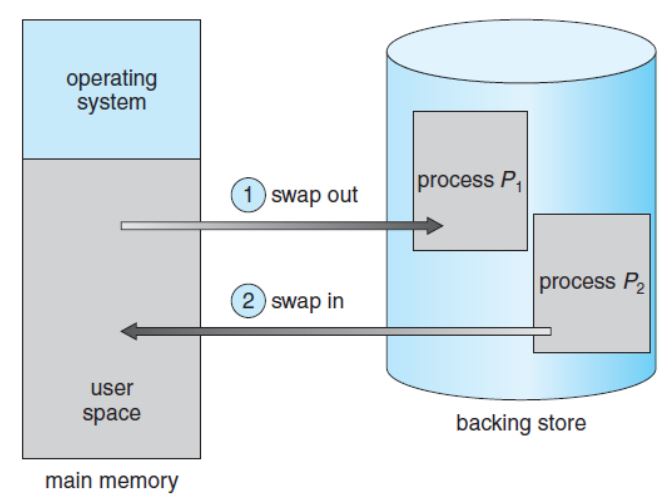
Swapping is constant by other factors:
- To swap a process, it should be completely idle.
- A process may be waiting for an I/O operation. If the I/O is asynchronously accessing the user memory for I/O buffers, then the process cannot be swapped.
Sr. No. | Logical Address | Physical Address |
1. | The address generated by the CPU | The address is seen by the memory unit |
2. | The set of all logical addresses generated by a program is a logical address space. | The set of all physical addresses corresponding to these logical addresses is physical address space. |
3. | Logical addresses (in the range 0 to max) | Physical addresses (in the range R 0 to R + max for a base value R) |
4. | Users can view the logical address of a program | Users can never view the Physical address of a program |
5. | Users can use the logical address to access the physical address | Users can indirectly access physical addresses but not directly |
6. | The logical address is variable hence will change with the system | The physical address of that object always remains constant. |
Memory Allocation
- Hole – block of available memory; holes of various sizes are scattered throughout memory.
- When a process arrives, it is allocated memory from a hole large enough to accommodate it.
- The operating system maintains information about:
- Allocated partitions
- Free partitions (hole)
- A set of holes of various sizes, is scattered throughout memory at any given time. When a process arrives and needs memory, the system searches this set for a hole that is large enough for this process.
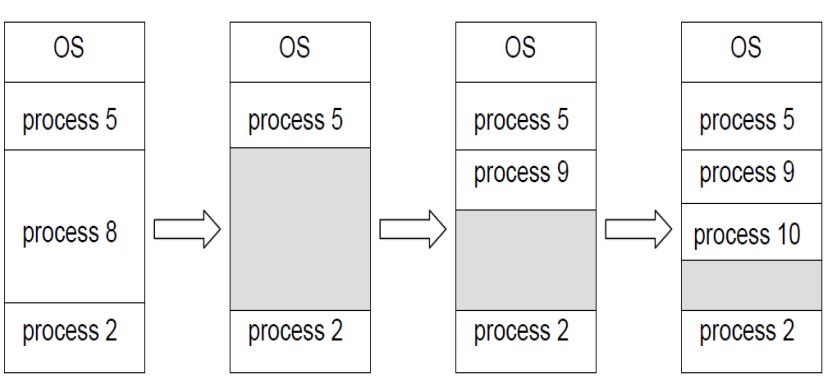
Memory management can be broadly classified into two types:
- Contiguous memory management
- Non-contiguous memory management
- Internal fragmentation can be described as when there is a left-over space that cannot be utilized further.
- External fragmentation takes place when there is enough space to satisfy the request of a process but it is not contiguous, so it cannot be utilized further.
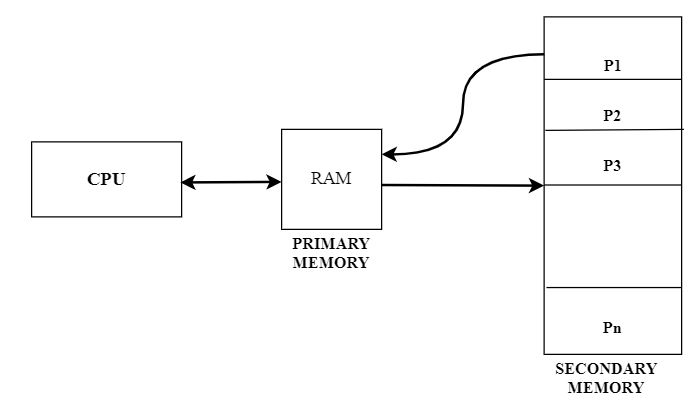
Contiguous memory management
The word contiguous
here refers to the shared allocation of a memory resource. The two important types of contiguous memory management are:
- Fixed partitioning
- Dynamic partitioning
Fixed partitioning
- Fixed-size partitioning is also called static partitioning.
- In fixed partitioning, the number of partitions is fixed.
- In fixed partitioning, the size of each partition may or may not be the same.
- In fixed partitioning, spanning is not allowed, which means that the entire process has to be allocated into a partition block. This also means that only a portion of the process cannot be allocated.
Limitations
- Internal fragmentation
- Limits the process size
- Limits the degree of multi-programming
- External fragmentation
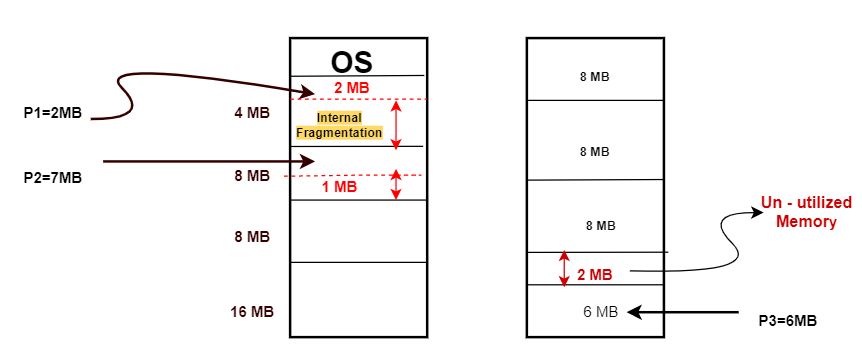
Dynamic partitioning
- Dynamic partitioning is a variable-size partitioning.
- In dynamic partitioning, the memory is allocated at run-time based on the requirement of processes.
- We usually consider the available block of memory as a hole.
- There is no internal fragmentation in dynamic partitioning.
- In dynamic partitioning, there is no limitation on the number of processes.
- The only limitation of dynamic partitioning is that it suffers from external Fragmentation.